Mobile SDKs
Verify individuals with a native iOS and Android experience.
Integrate the Persona Inquiry flow directly into your Android or iOS app with our native SDKs. Get up and running with a theme-able Inquiry flow with a few lines of code. If you're ready to get started, check out our technical documentation for Android, iOS, and React Native . See below for an example.
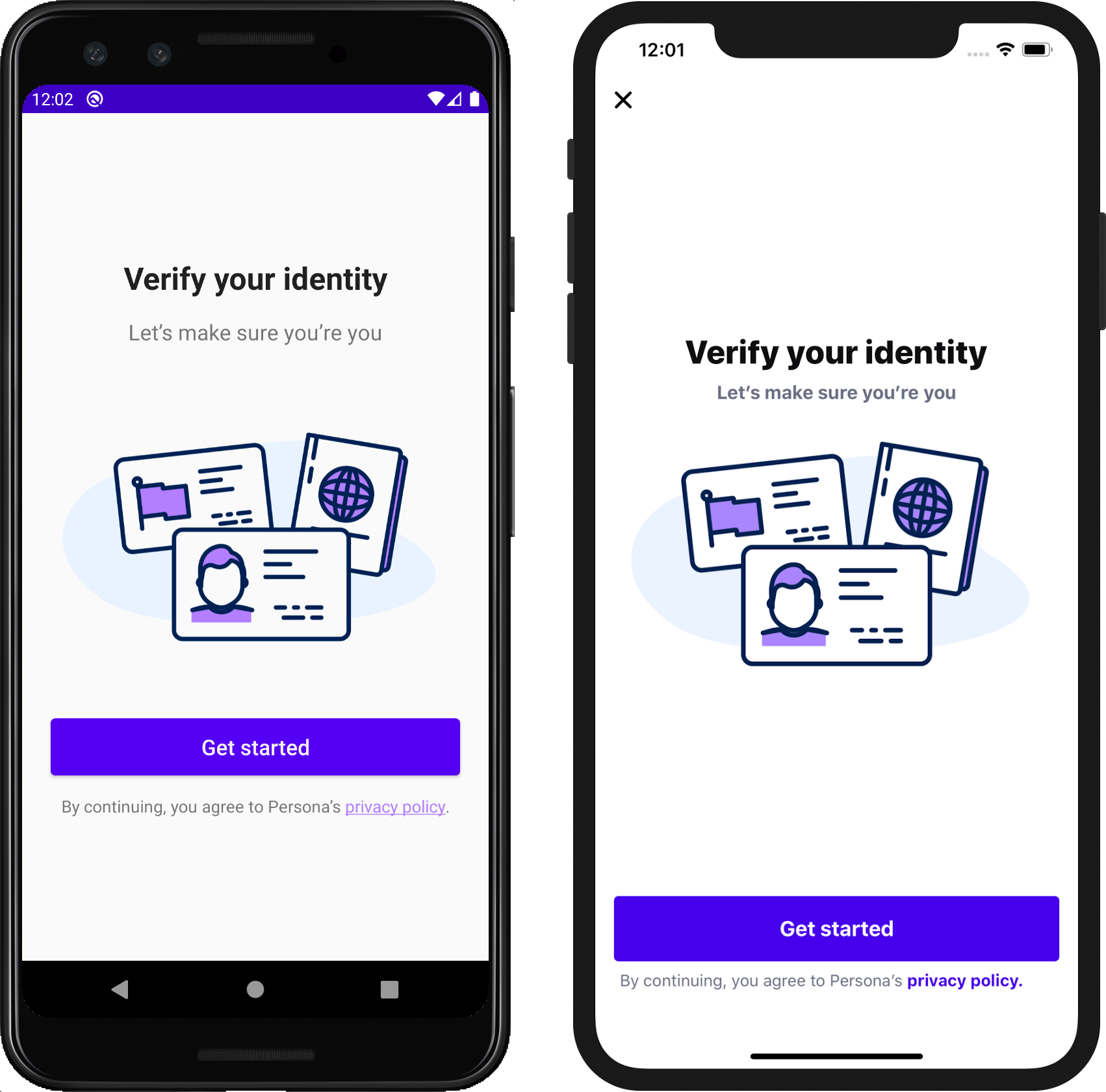
Example Native Android and iOS usage
// to launch the Inquiry flow
Inquiry.Builder("tmpl_JAZjHuAT738Q63BdgCuEJQre")
.environment(Environment.SANDBOX)
.build()
.start(this, VERIFY_REQUEST_CODE)
// overwriting the Activity#onActivityResult
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == VERIFY_REQUEST_CODE) {
when(Inquiry.onActivityResult(data)) {
is Inquiry.Response.Success -> {
// ✅ Inquiry succeeded
}
Inquiry.Response.Cancel -> {
// ⏏️ Inquiry cancelled by user
}
is Inquiry.Response.Failure -> {
// ❌ Inquiry failed
}
is Inquiry.Response.Error -> {
// ⚠️ Inquiry errored
}
}
}
}
class ViewController: UIViewController, InquiryDelegate {
// This is hooked up to a button which starts the flow
@IBAction func buttonTapped(_ sender: UIButton) {
let config = InquiryConfiguration(templateId: "tmpl_JAZjHuAT738Q63BdgCuEJQre")
// Create the inquiry with the view controller
// as the delegate and presenter.
Inquiry(config: config, delegate: self).start(from: self)
}
// MARK: - Inquiry Delegate Methods
func inquirySuccess(inquiryId: String, attributes: Attributes, relationships: Relationships) {
// ✅ Inquiry succeeded
}
func inquiryCancelled() {
// ⏏️ Inquiry cancelled by user
}
func inquiryFailed(inquiryId: String, attributes: Attributes, relationships: Relationships) {
// ❌ Inquiry failed
}
func inquiryError(_ error: Error) {
// ⚠️ Inquiry errored
}
}
Updated almost 5 years ago
Next steps
Begin integrating with the Android and iOS SDKs.