Serialization
Data
Requests and responses follow the JSON:API specification, using JSON-encoded bodies. List, fetch, and action endpoints do not require the request to contain a body. Responses contain a single JSON-encoded object with the following keys:
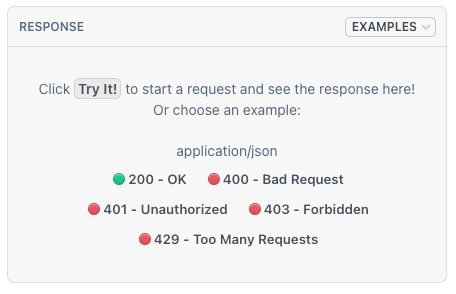
Related resources
Under data
, the relationships
key will include information about all resources that are related somehow to the primary resource. For example, an inquiry resource will have a relationship sessions
that references all inquiry-session
resources associated with the given inquiry. The keys within relationships
are the canonical names for that relationship.
Relationships can be one-to-one or one-to-many. The value of the data
key for any given relationship will be an array for a one-to-many relationship or a hash for a one-to-one relationship with the primary resource.
relationships
will only include the type
and id
of the related resource. You can think of type
and id
as a composite key that uniquely references resource objects in another part of the response.
Inclusion of related resources
By default, each API will return fully serialized representations of some/none/all of its related resources under the included
key. You can use the include
query parameter to override this default behavior and request which relationships you’d like to have returned in included
(or none). Note that this parameter is not allowed for “list all” endpoints.
The value of the include
parameter is a comma-separated (U+002C COMMA, “,”) list of relationship paths. A relationship path is a dot-separated (U+002E FULL-STOP, “.”) list of relationship names. An empty value indicates that no related resources should be returned (included
will still be present in the response and its value an empty array).
Anything that is defined in the relationships
array for a resource can be requested with the include
parameter. If you do not see the desired object in the relationships
array for the resource returned by an endpoint, you cannot use include
to retrieve it.
Please note that at most one level of dot-separated nesting is allowed in the include
parameter. That is, ?include=foo.bar
is allowed, but requests with ?include=foo.bar.baz
are not, and will result in a 400
response.
Take the retrieve an inquiry API as an example:
Sparse fieldsets
By default, retrieving a resource via API will return fully serialized representations of all attributes
(including fields
) under the data
key. You can use sparse fieldsets to override this default behavior and request only the attributes that you’d like returned.
In essence, sparse fieldsets allow you to request only specific attributes from the resources returned by Persona’s APIs. The benefits of using sparse fieldsets include:
- Reduced data transfer - By requesting only the fields that your system needs, you can eliminate the risk surface around passing unintended personal information or other data into your system.
- Improved response time - By specifying specific fields in your API call, Persona is able to retrieve exactly what matters as opposed to every field related to the resource and return it to your system quickly.
Sparse syntax
Sparse fieldsets are specified using the fields[TYPE]
query parameter, where TYPE
is the singular resource type (e.g. inquiry
, verification
, transaction
) from which you’d like to select specific attributes. The value of the fields
parameter is a comma-separated (U+002C COMMA, “,”) list of attribute names. Attribute names are any keys present under the nested key data → attributes.
Some Persona resources (like Inquiries and Transactions) will include fields
nested under data
→ attributes
. As fields can be extensive and contain PII, it is common for developers to only want to retrieve specific keys within fields
. You can request only specific fields to be returned by using a comma-separated (U+002C COMMA, “,”) list of field names, prefixed with the attribute name it is nested under (e.g.fields.name-first
, fields.name-last
, fields.address-street-1
) as the value of the fields[TYPE]
query parameter.
Both attribute and field names can be used simultaneously in the fields[TYPE]
query parameter value list.
In the following examples of retrieving an Inquiry, you can see what use of sparse fieldsets can do to simplify API responses.
Sparse fieldsets with included resources
You can specify sparse fieldsets for included resources as well. For example, you might want to retrieve a Verification status linked to a specific Inquiry. To do this, you must use the include query parameter to include
the verification
resource. Then, you can set verification as the resource type and status as a field shown in the example below: